Getting Started With Arduino
Arduino Programming
I have an old Arduino Duemilanove board that I bought a long time ago and have since neglected.
This is a very old board, first released in 2009 and no longer available except maybe on Ebay.
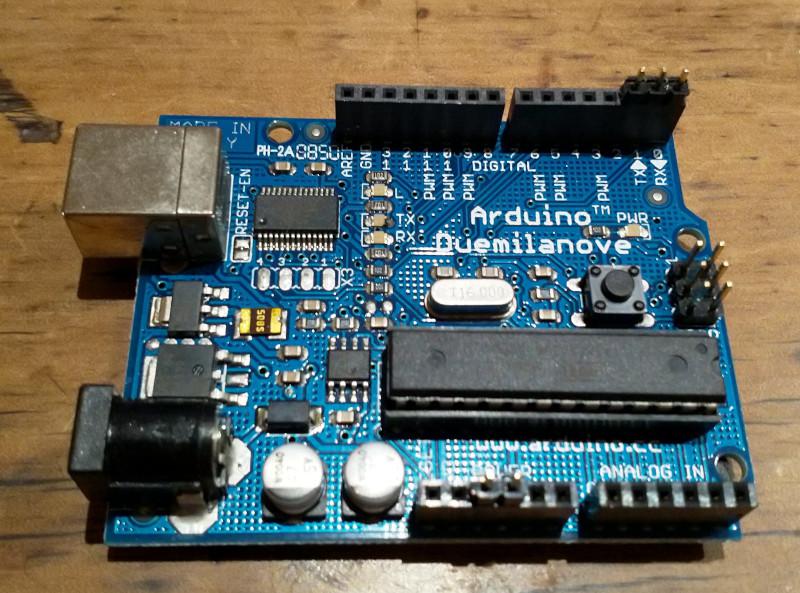
To get started with using this microcontroller you have to download the Arduino IDE.
- Arduino IDE
-
Download the Arduino IDE and install. It is likely that you will have to add your user account to the dialout group and log in again. I also had to make the
/dev/ttyUSB0
device group read+write-able. There may be other ways to do this but I used reliable oldchmod g+rw /dev/ttyUSB0
.
A good hello world project is to upload the blink sketch. This makes the onboard LED flash, which confirms that you are able to do something with the microcontroller.
I modified the sketch slightly to make the LED stay on for three seconds, just to be sure .
/* Blink Turns an LED on for three seconds, then off for one second, repeatedly. http://www.arduino.cc/en/Tutorial/Blink */ // the setup function runs once when you press reset or power the board void setup() { // initialize digital pin LED_BUILTIN as an output. pinMode(LED_BUILTIN, OUTPUT); } // the loop function runs over and over again forever void loop() { digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level) delay(3000); // wait for a second digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW delay(1000); // wait for a second }
Click the Verify (tick mark) and Upload (right arrow) buttons and the sketch should upload to the board and the LED should start blinking.
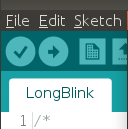
Trouble
I had a lot of trouble getting this to work at all. There are a lot of basic things that you have to get right.
As mentioned, fix group access permissions to the USB device.
Make sure you have selected the right board from the Tools > Board menu, it won't be auto-detected for you.
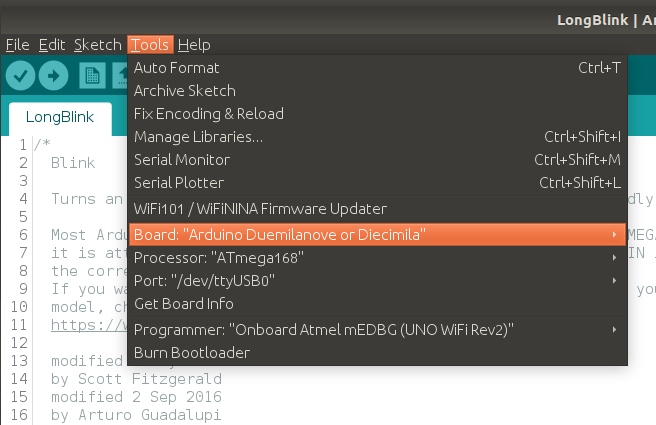
Select the right processor from the Tools > Processor menu. It might help to have a close look at the chip on the board to work out which one it is.
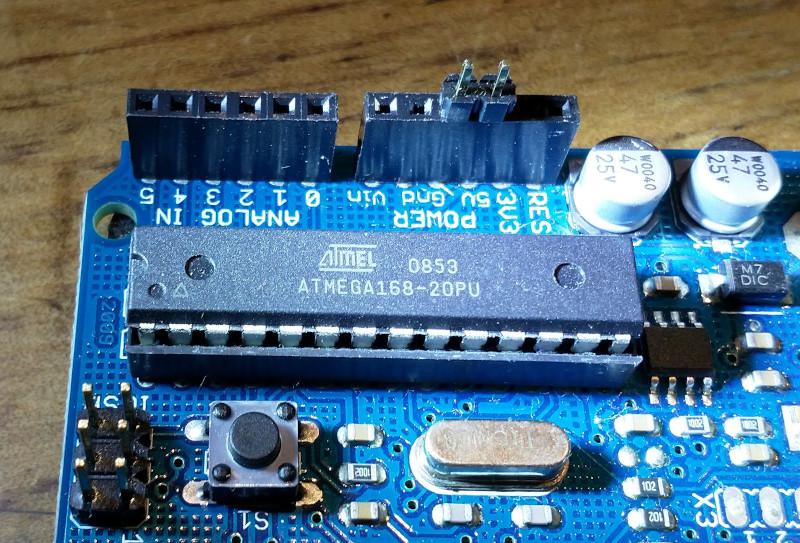
If this all fails, you can turn on additional debugging output from File > Preferences > Show Verbose Output and start searching Stack Overflow.
Comments
Comments powered by Disqus