Arduino + Python + LED Matrix
An LED Matrix
I went to Jaycar, looking for a WiFi module as I was thinking I'll need one for my e-paper calendar project. I walked out with a number of things including a Duinotech Uno with WiFi and an 8x8 red LED Matrix.
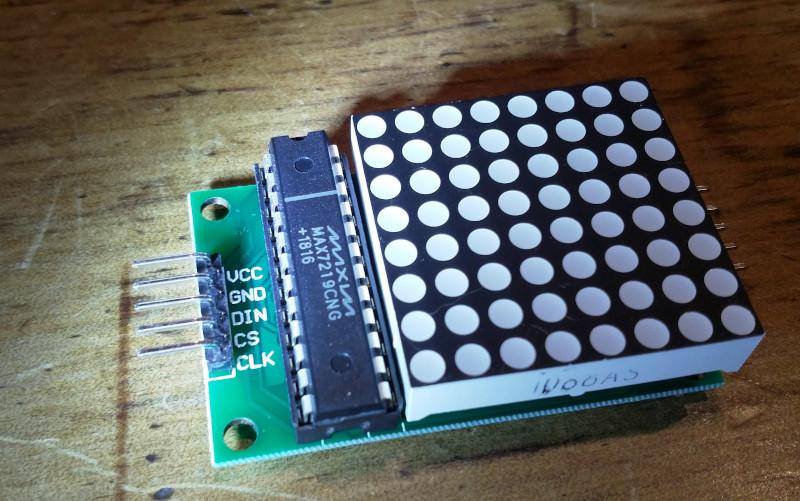
OMG The Pins
This is a little lesson about reading the documentation. I spent hours trying to upload a blink sketch to the Uno. I tried all the boards available in the IDE. I loaded more boards using the IDE Boards Manager. I did rend my clothes and tear my hair. Finally I looked at the Jaycar site to try to find some howto stories.
On the product page linked above I found a note:
Please note: you must upload code to both processors then configure the switches for them to talk to each other
And a manual, and in the manual I found found some very clear instructions explaining that you have to set some dip switches on the board in order to program the Arduino MCU.
As they are two separate processors, you must upload code to both processors for them to function. You can set this by configuring the dip switches as per the table below, so that the USB/programmer can communicate between both processors individually. Once you have finished programming, configure the dip switches so both of the processors can communicate with each other to send messages back and forth.
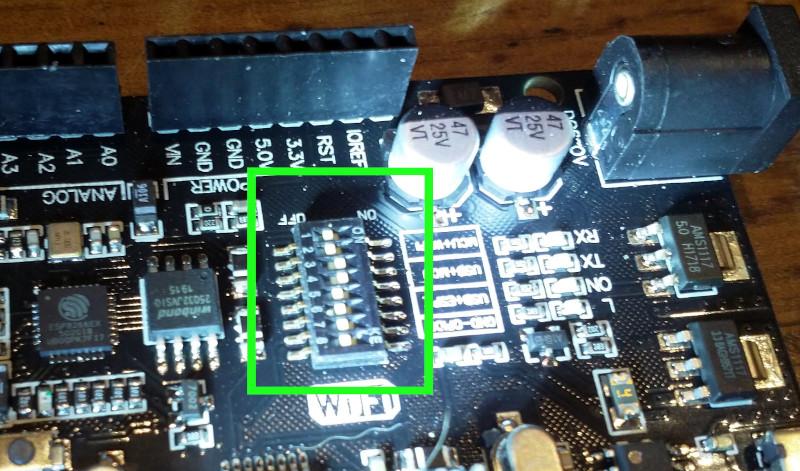
For the moment I am not using the wireless functionality, so I've just set the dip switches so that I can program the Arduino MCU.
- Interactivity
-
To work with the Arduino you have to be able to program it in C++. But the compile, upload, run loop of C++ can be daunting and time consuming. A more interactive programming cycle can give you more freedom to try out ideas, let you be more creative and is generally helpful for learning. I do a lot of programming in Python and I wanted to see if there was some practical way of working with the Arduino with Python.
- Firmata
-
Firmata is a protocol for communicating with microcontrollers from software on a host computer. The protocol can be implemented in firmware on any microcontroller architecture as well as software on any host computer software package.
You load a general purpose sketch called StandardFirmata (or StandardFirmataPlus in this example) on the Arduino board and then use the host computer exclusively to interact with the Arduino board. In the Arduino IDE the Firmata sketches can be found in File -> Examples -> Firmata.
- PyMata
-
PyMata is a Python library for interacting with an system running Firmata. The library has three flavours, the most straightforward of which lets you interact directly with the Arduino from the python interpreter.
Firmata Hello World
A basic Firmata hello world is to turn the on-board LED on and off.
First you load the Firmata sketch onto the Arduino. I tried StandardFirmataPlus. I quickly became apparent that this would not run on the Duemilanove. The only Firmata sketch the would was the OldStandardFirmata sketch. The python library, however, would not connect to this. In the end I got StandardFirmataPlus running with the Uno.
The python library was easy to install with pip install pymata-aio
Turning the on board LED on and off:
from pymata_aio.pymata3 import PyMata3 from pymata_aio.constants import Constants board = PyMata3(arduino_wait=5) BOARD_LED = 13 def setup(): board.set_pin_mode(BOARD_LED, Constants.OUTPUT) def loop(): print("LED On") board.digital_write(BOARD_LED, 1) board.sleep(1.0) print("LED Off") board.digital_write(BOARD_LED, 0) board.sleep(1.0) setup() while True: loop()
MaxMatrix
But why just light up one LED when you can use 64? I did some work with the C++ MaxMatrix library and then ported it to python so I could drive the matrix from the python command line.
- Wiring The LED
-
The LED is easy to wire. The VCC and GND pins should be connected to 5V and GND. The remaining pins can be connected to any digital pins on the board. They just have to be correctly identified when using the software.
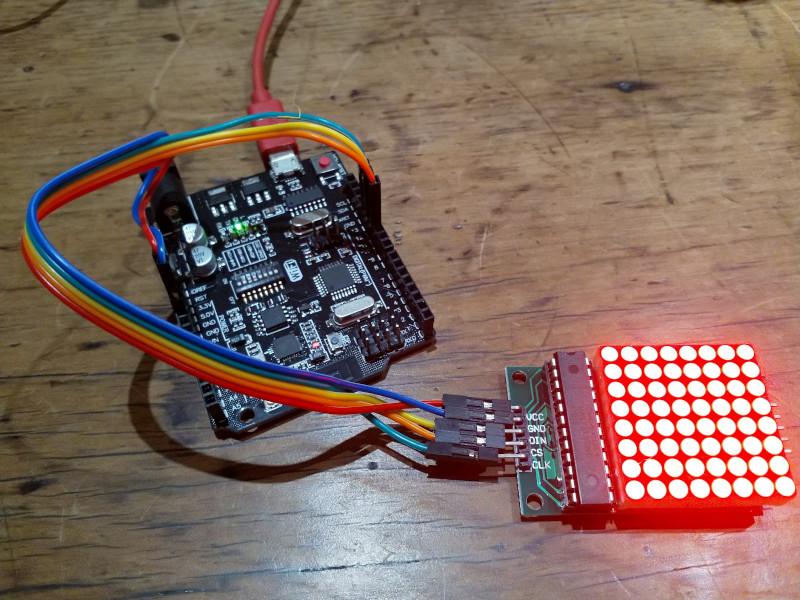
One nice thing about driving the Arduino interactively is that you don't need to worry about memory as much. I was able to add an additional method to the library to load a Dwarf Fortress tileset and use this as a convenient source of high quality 8x8-bit ascii graphics. I chose the Potash tileset
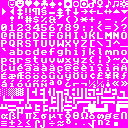
Some sample code:
from pymata_aio.pymata3 import PyMata3 from pymata_aio.constants import Constants import maxmatrix board = PyMata3(arduino_wait=5) # I wired the LED to 11, 12 and 13 DIN, CLK, CS = 12, 13, 11 mm = maxmatrix.MaxMatrix(board, DIN, CS, CLK) mm.set_column(0, 0b1011011)
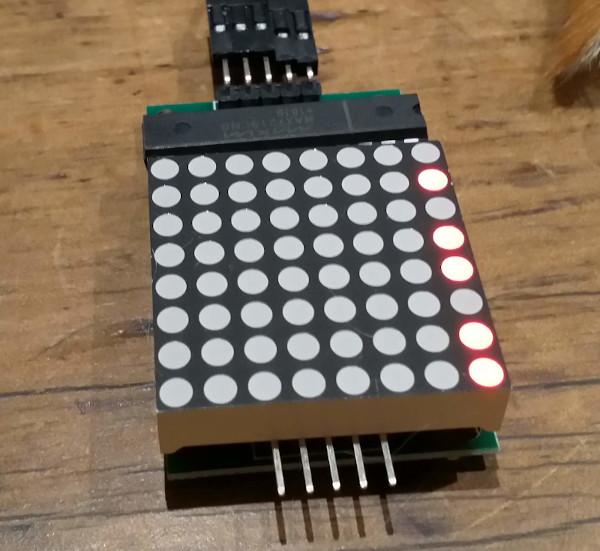
Working with sprites from a tileset:
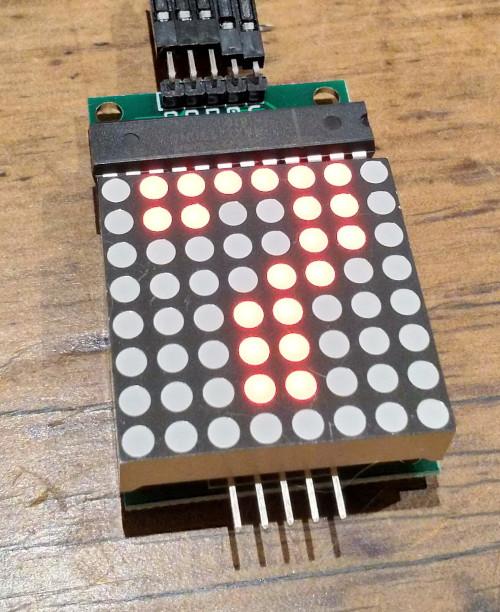
maxmatrix.py
Full code listing of my python port of the MaxMatrix library.
As I only have the one LED I haven't dealt with the parts of the MaxMatrix library that handle with multiple daisy-chained LEDs.
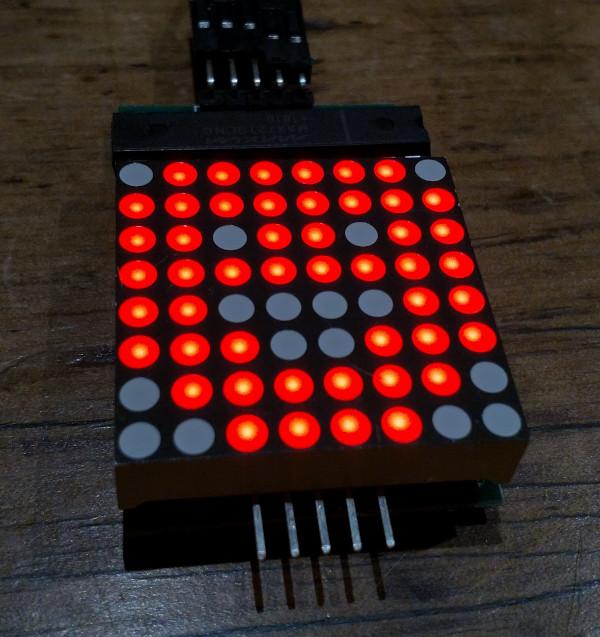
Comments
Comments powered by Disqus